Polymorphism in Java: Meaning and its Types

Polymorphism is a fancy-sounding word that really just means “many shapes.” And in the world of Java programming, it refers to the ability of objects to take on different forms depending on the context in which they are used.
You might say that Polymorphism is like a chameleon, changing its colors and shapes to fit into different environments. Or, if you’re feeling particularly silly, you could say that polymorphism is like a superhero with a secret identity, able to transform into different personas depending on the situation.
Of course, these comparisons are all just metaphors, and polymorphism in Java is a bit more complicated than changing colors or donning a cape. But hey, if it helps you remember the concept, then who am I to judge?
Click to compare Java Course by Coursera, Udemy, Udacity and others.
Topics to be covered in this blog:
- What is Polymorphism?
- Importance of Polymorphism in Java
- Use of Polymorphism in Java in Different Industry
- Importance of Polymorphism in Java
- Types of Polymorphism in Java
- Compile-time vs Runtime Polymorphism
- Benefits of Polymorphism
Key Highlights: Polymorphism in Java
- Polymorphism: Java feature allowing multiple object forms
- Treats different objects as the same type
- Two types: Compile-time and Runtime Polymorphism
- Compile-time: method overloading (same name, different parameters)
- Runtime: method overriding (subclass provides own method implementation)
- Enhances code flexibility and reusability
- Used in object-oriented programming for inheritance and interfaces
Best-suited Java courses for you
Learn Java with these high-rated online courses
What is Polymorphism?
Polymorphism is like a Swiss Army Knife, with multiple tools in one object.
Polymorphism in Java is like a Swiss Army Knife – it’s a single tool that can take on many different forms, depending on the task at hand. Just like a Swiss Army Knife has different tools for different purposes, a Java object can take on different behaviors depending on the context in which it is used.
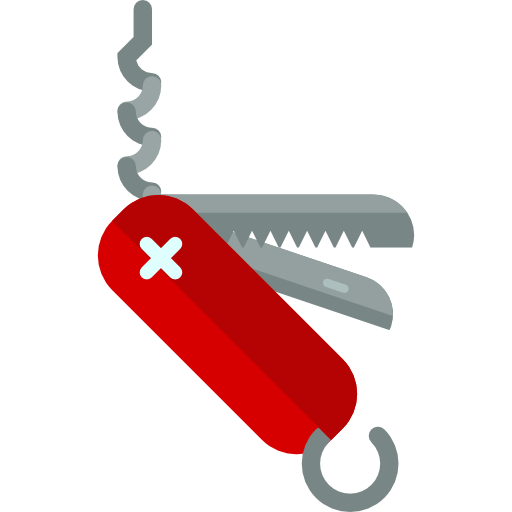
- Polymorphism means having multiple forms.
- Polymorphism – “poly” meaning many and “morph” meaning forms.
Simpler example, a woman can have multiple roles, such as a data analyst, a software engineer, a tech blogger, a designer, etc depending on the profession. This ability to take on different roles or forms is what we call polymorphism.
For more, Read: OOPs Concepts in Java.
What is Polymorphism in Java?
Polymorphism in Java can be explained in simple terms as the ability of different objects to be treated as if they were the same type of object. It allows developers to write code that can work with different types of objects without knowing their specific types, as long as they share a common interface or inheritance relationship. This means that a method can be called on an object, and different objects can respond to that method in different ways, depending on their specific implementation. Polymorphism helps make code more flexible, extensible, and maintainable.
Read the code:
NOTE: Below code is in a readable and easy to understand format. Try to read the code comment (//_) and follow the code to understand the implementation of Java.
// Define a superclass called "Animal"public class Animal { // Define a method called "makeSound" public void makeSound() { System.out.println("The animal makes a sound"); }}
// Define a subclass called "Dog" that inherits from "Animal"public class Dog extends Animal { // Override the "makeSound" method to make the dog bark public void makeSound() { System.out.println("The dog barks"); }}
// Define a subclass called "Cat" that inherits from "Animal"public class Cat extends Animal { // Override the "makeSound" method to make the cat meow public void makeSound() { System.out.println("The cat meows"); }}
// Define a main class to run the programpublic class Main { public static void main(String[] args) { // Create an instance of the "Animal" class Animal animal1 = new Animal(); // Create an instance of the "Dog" class Animal animal2 = new Dog(); // Create an instance of the "Cat" class Animal animal3 = new Cat();
// Call the "makeSound" method on the "Animal" object animal1.makeSound(); // The animal makes a sound // Call the "makeSound" method on the "Dog" object animal2.makeSound(); // The dog barks // Call the "makeSound" method on the "Cat" object animal3.makeSound(); // The cat meows }}
GIVE IT A TRY!
Problem Statement: Create a virtual robot in Java and give it the ability to perform different tasks depending on its “mode“. For example, in “cleaning mode“, the robot might clean the floor, while in “entertainment mode“, it might play music or tell jokes. This is an example of polymorphism in action.
What is Shiksha Online? How can it help you master your skillset in Java?
“If you’re a coding enthusiast who’s eager to learn and add Polymorphism in Java to your skillset, you’re in luck. Because at Shiksha Online, we’ve got you covered! We understand that choosing the right course can be overwhelming. That’s why we aggregate courses from the best e-learning vendors like Coursera, Udemy, and Udacity. This means you can easily compare and find the perfect course for your learning style.”
Sshhh: Here’s the list of 100+ FREE courses in Java
Our platform boasts a user-friendly interface and comprehensive course listings, so you can be confident you’re getting the best education possible. Whether you’re a beginner or an expert, we have courses tailored to suit your needs. So, why wait? Start your learning journey with Shiksha Online today and take the first step towards mastering Polymorphism in Java!
Use of Polymorphism in Java in Different Industry
Industry | Product/Software | Polymorphism Example |
---|---|---|
E-commerce | Online Shopping Platform | Different product types (clothes, electronics) with unique addToCart and checkAvailability implementations |
Finance | Banking Software | Diverse account types (savings, checking) with customized withdraw and transfer methods |
Healthcare | Hospital Management System | Various patient types (inpatient, outpatient) with tailored calculateBill and scheduleAppointment methods |
Video Games | Game Engine | Distinct game characters with unique attack and move implementations |
Transportation | Ride-Hailing App | Different vehicle types (car, bike) with specialized calculateFare and estimateArrivalTime methods |
Importance of Polymorphism in Java
Principle | Example | Description |
---|---|---|
Code Reusability | Inheritance | A Dog class inherits speak() from Animal class, and can override it to bark while still using the inherited method. |
Flexibility | Interfaces | A Flying interface implemented by Bird and Plane classes lets both have a fly() method and be treated as the same class. |
Abstraction | Abstract Classes | Animal class defines abstract eat() method, which must be implemented by subclasses like Dog and Cat. |
Encapsulation | Polymorphism & Interfaces | A Car class has a start() method that internally calls the igniteEngine() method of an Engine class without exposing Engine details. |
Extensibility | Method Overriding | Triangle class overrides calculateArea() from Shape class to calculate area differently than a Rectangle class, which also inherits from Shape class. |
Types of Polymorphism in Java
Polymorphism is of two types:
Difference between Compile Time and Run Time Polymorphism
Compile-time polymorphism is achieved through method overloading, where methods with the same name but different parameters are defined in a class. On the other hand, runtime polymorphism is achieved through method overriding, where a subclass provides its own implementation of a method that is already defined in its superclass. Compile-time polymorphism occurs during compile-time, while runtime polymorphism occurs during runtime. The selection of the appropriate method also differs, with the compiler selecting the appropriate method for compile-time polymorphism based on the arguments passed to it, while the JVM selects the appropriate method for runtime polymorphism based on the type of the object being referred to.
Parameter | Compile-time Polymorphism | Runtime Polymorphism |
---|---|---|
Definition | Method overloading | Method overriding |
Occurs | During compile-time | During runtime |
Parameters | Different number, order, or type of parameters | Same number and type of parameters |
Example | public void print(int x) and public void print(String s) in the same class | Subclass provides its own implementation of a method already defined in the superclass |
Syntax | Two or more methods with the same name and different parameters | Method in a subclass has the same name, return type, and parameter list as a method in the superclass |
Selection | Compiler selects the appropriate method based on the arguments passed to it | JVM selects the appropriate method at runtime based on the type of the object being referred to |
Goal | To provide different behavior of methods based on the input arguments | To provide a specific behavior to a method that is common to all objects of a particular class hierarchy |
Type | Static polymorphism | Dynamic polymorphism |
Compile-time Polymorphism (Static Binding)
Compile-time polymorphism is like having different boxes labeled with the same name, but with different contents inside. Depending on what you need, you can choose the box with the specific contents you want. In the same way, when you have multiple methods with the same name in a Java class, but with different parameters, the compiler chooses the right method to execute based on the parameters you pass to it. It’s like choosing the box with the specific contents you need.
Method Overloading is a type of static or compile-time polymorphism. Why?
Method overloading means having multiple methods with the same name but different parameters. For example, there could be a method named “add” that takes two integers as parameters, and another method named “add” that takes two doubles as parameters.
When you call an overloaded method, the compiler determines which version of the method to call based on the number, order, and types of the parameters that you pass to it. This decision is made by the compiler at compile-time, before the program runs, and is fixed.
This is why method overloading in Java is an example of static or compile-time polymorphism. And since the binding between the method call and the method to be executed is done at compile-time and is fixed, it is also an example of static binding.
Example:
public class MathUtils { // Define a method to add two integers public int add(int a, int b) { return a + b;A } // Define a method to add three integers public int add(int a, int b, int c) { return a + b + c; } // Define a method to add two doubles public double add(double a, double b) { return a + b; }}
// Call the methodsMathUtils math = new MathUtils();System.out.println(math.add(2, 3)); // Output: 5System.out.println(math.add(2, 3, 4)); // Output: 9System.out.println(math.add(2.5, 3.5)); // Output: 6.0
In this example, we have defined three methods called “add” in the “MathUtils” class. Each of these methods has a different set of parameters: one takes two integers, one takes three integers, and one takes two doubles.
When we call the “add” method on an instance of the “MathUtils” class, Java determines which version of the method to call based on the number and types of arguments that we pass to it. This is an example of method overloading, where we have multiple methods with the same name but different parameters.
Constructor Overloading
If more than one constructor is declared inside a class with different parameters is called “Constructor Overloading.” FYI, a constructor is a special member function of a class with the same name as the class name. Whenever an object of a class is created using a new keyword, it invokes a constructor of that class.
Example:
<pre class="java" style="font-family:monospace"> <span style="color: #000000;font-weight: bold"> class <span style="color: #003399"> Rectangle <span style="color: #009900"> { <span style="color: #000066;font-weight: bold"> double length <span style="color: #339933"> ; <span style="color: #000066;font-weight: bold"> double breadth <span style="color: #339933"> ; <span style="color: #003399"> Rectangle <span style="color: #009900"> ( <span style="color: #009900"> ) <span style="color: #009900"> { length <span style="color: #339933"> = <span style="color: #cc66cc"> 10 <span style="color: #339933"> ; breadth <span style="color: #339933"> = <span style="color: #cc66cc"> 20 <span style="color: #339933"> ; <span style="color: #009900"> } <span style="color: #003399"> Rectangle <span style="color: #009900"> ( <span style="color: #000066;font-weight: bold"> double l, <span style="color: #000066;font-weight: bold"> double b <span style="color: #009900"> ) <span style="color: #009900"> { length <span style="color: #339933"> = l <span style="color: #339933"> ; breadth <span style="color: #339933"> = b <span style="color: #339933"> ; <span style="color: #009900"> } <span style="color: #000066;font-weight: bold"> double calculateArea <span style="color: #009900"> ( <span style="color: #009900"> ) <span style="color: #009900"> { <span style="color: #000000;font-weight: bold"> return length <span style="color: #339933"> *breadth <span style="color: #339933"> ; <span style="color: #009900"> } <span style="color: #009900"> } <span style="color: #000000;font-weight: bold"> class Rectangle_Main <span style="color: #009900"> { <span style="color: #000000;font-weight: bold"> public <span style="color: #000000;font-weight: bold"> static <span style="color: #000066;font-weight: bold"> void main <span style="color: #009900"> ( <span style="color: #003399"> String <span style="color: #009900"> [ <span style="color: #009900"> ] args <span style="color: #009900"> ) <span style="color: #009900"> { <span style="color: #000066;font-weight: bold"> double area, area1 <span style="color: #339933"> ; <span style="color: #003399"> Rectangle myrec1 <span style="color: #339933"> = <span style="color: #000000;font-weight: bold"> new <span style="color: #003399"> Rectangle <span style="color: #009900"> ( <span style="color: #009900"> ) <span style="color: #339933"> ; area1 <span style="color: #339933"> = myrec1. <span style="color: #006633"> calculateArea <span style="color: #009900"> ( <span style="color: #009900"> ) <span style="color: #339933"> ; <span style="color: #003399"> System. <span style="color: #006633"> out. <span style="color: #006633"> println <span style="color: #009900"> ( <span style="color: #0000ff"> "The area of Rectangle1: " <span style="color: #339933"> +area1 <span style="color: #009900"> ) <span style="color: #339933"> ; <span style="color: #003399"> Rectangle myrec <span style="color: #339933"> = <span style="color: #000000;font-weight: bold"> new <span style="color: #003399"> Rectangle <span style="color: #009900"> ( <span style="color: #cc66cc"> 41, <span style="color: #cc66cc"> 56 <span style="color: #009900"> ) <span style="color: #339933"> ; area <span style="color: #339933"> = myrec. <span style="color: #006633"> calculateArea <span style="color: #009900"> ( <span style="color: #009900"> ) <span style="color: #339933"> ; <span style="color: #003399"> System. <span style="color: #006633"> out. <span style="color: #006633"> println <span style="color: #009900"> ( <span style="color: #0000ff"> "The area of Rectangle2: " <span style="color: #339933"> +area <span style="color: #009900"> ) <span style="color: #339933"> ; <span style="color: #009900"> } <span style="color: #009900"> } </span style="color: #009900"> </span style="color: #009900"> </span style="color: #339933"> </span style="color: #009900"> </span style="color: #339933"> </span style="color: #0000ff"> </span style="color: #009900"> </span style="color: #006633"> </span style="color: #006633"> </span style="color: #003399"> </span style="color: #339933"> </span style="color: #009900"> </span style="color: #009900"> </span style="color: #006633"> </span style="color: #339933"> </span style="color: #339933"> </span style="color: #009900"> </span style="color: #cc66cc"> </span style="color: #cc66cc"> </span style="color: #009900"> </span style="color: #003399"> </span style="color: #000000;font-weight: bold"> </span style="color: #339933"> </span style="color: #003399"> </span style="color: #339933"> </span style="color: #009900"> </span style="color: #339933"> </span style="color: #0000ff"> </span style="color: #009900"> </span style="color: #006633"> </span style="color: #006633"> </span style="color: #003399"> </span style="color: #339933"> </span style="color: #009900"> </span style="color: #009900"> </span style="color: #006633"> </span style="color: #339933"> </span style="color: #339933"> </span style="color: #009900"> </span style="color: #009900"> </span style="color: #003399"> </span style="color: #000000;font-weight: bold"> </span style="color: #339933"> </span style="color: #003399"> </span style="color: #339933"> </span style="color: #000066;font-weight: bold"> </span style="color: #009900"> </span style="color: #009900"> </span style="color: #009900"> </span style="color: #009900"> </span style="color: #003399"> </span style="color: #009900"> </span style="color: #000066;font-weight: bold"> </span style="color: #000000;font-weight: bold"> </span style="color: #000000;font-weight: bold"> </span style="color: #009900"> </span style="color: #000000;font-weight: bold"> </span style="color: #009900"> </span style="color: #009900"> </span style="color: #339933"> </span style="color: #339933"> </span style="color: #000000;font-weight: bold"> </span style="color: #009900"> </span style="color: #009900"> </span style="color: #009900"> </span style="color: #000066;font-weight: bold"> </span style="color: #009900"> </span style="color: #339933"> </span style="color: #339933"> </span style="color: #339933"> </span style="color: #339933"> </span style="color: #009900"> </span style="color: #009900"> </span style="color: #000066;font-weight: bold"> </span style="color: #000066;font-weight: bold"> </span style="color: #009900"> </span style="color: #003399"> </span style="color: #009900"> </span style="color: #339933"> </span style="color: #cc66cc"> </span style="color: #339933"> </span style="color: #339933"> </span style="color: #cc66cc"> </span style="color: #339933"> </span style="color: #009900"> </span style="color: #009900"> </span style="color: #009900"> </span style="color: #003399"> </span style="color: #339933"> </span style="color: #000066;font-weight: bold"> </span style="color: #339933"> </span style="color: #000066;font-weight: bold"> </span style="color: #009900"> </span style="color: #003399"> </span style="color: #000000;font-weight: bold"> </pre class="java" style="font-family:monospace">
Output:



Run-time Polymorphism (Dynamic Binding)
Run-time polymorphism or late binding polymorphism, determined at run time depicts using overriding.
Method Overriding
Method Overriding is redefining a superclass method with the same name, data types, and parameters in a subclass.
Example:
<pre class="java" style="font-family:monospace"> <span style="color: #000000;font-weight: bold"> class <span style="color: #003399"> Rectangle
<span style="color: #009900"> { <span style="color: #000066;font-weight: bold"> double length <span style="color: #339933"> ; <span style="color: #000066;font-weight: bold"> double breadth <span style="color: #339933"> ; <span style="color: #003399"> Rectangle <span style="color: #009900"> ( <span style="color: #009900"> ) <span style="color: #009900"> { length <span style="color: #339933"> = <span style="color: #cc66cc"> 10 <span style="color: #339933"> ; breadth <span style="color: #339933"> = <span style="color: #cc66cc"> 20 <span style="color: #339933"> ; <span style="color: #009900"> } <span style="color: #003399"> Rectangle <span style="color: #009900"> ( <span style="color: #000066;font-weight: bold"> double l, <span style="color: #000066;font-weight: bold"> double b <span style="color: #009900"> ) <span style="color: #009900"> { length <span style="color: #339933"> = l <span style="color: #339933"> ; breadth <span style="color: #339933"> = b <span style="color: #339933"> ; <span style="color: #009900"> } <span style="color: #000066;font-weight: bold"> double calculateArea <span style="color: #009900"> ( <span style="color: #009900"> ) <span style="color: #009900"> { <span style="color: #000000;font-weight: bold"> return length <span style="color: #339933"> *breadth <span style="color: #339933"> ; <span style="color: #009900"> }
<span style="color: #009900"> }
<span style="color: #000000;font-weight: bold"> class Rectangle_Main <span style="color: #009900"> { <span style="color: #000000;font-weight: bold"> public <span style="color: #000000;font-weight: bold"> static <span style="color: #000066;font-weight: bold"> void main <span style="color: #009900"> ( <span style="color: #003399"> String <span style="color: #009900"> [ <span style="color: #009900"> ] args <span style="color: #009900"> ) <span style="color: #009900"> { <span style="color: #000066;font-weight: bold"> double area, area1 <span style="color: #339933"> ; <span style="color: #003399"> Rectangle myrec1 <span style="color: #339933"> = <span style="color: #000000;font-weight: bold"> new <span style="color: #003399"> Rectangle <span style="color: #009900"> ( <span style="color: #009900"> ) <span style="color: #339933"> ; area1 <span style="color: #339933"> = myrec1. <span style="color: #006633"> calculateArea <span style="color: #009900"> ( <span style="color: #009900"> ) <span style="color: #339933"> ; <span style="color: #003399"> System. <span style="color: #006633"> out. <span style="color: #006633"> println <span style="color: #009900"> ( <span style="color: #0000ff"> "The area of Rectangle1: " <span style="color: #339933"> +area1 <span style="color: #009900"> ) <span style="color: #339933"> ; <span style="color: #003399"> Rectangle myrec <span style="color: #339933"> = <span style="color: #000000;font-weight: bold"> new <span style="color: #003399"> Rectangle <span style="color: #009900"> ( <span style="color: #cc66cc"> 41, <span style="color: #cc66cc"> 56 <span style="color: #009900"> ) <span style="color: #339933"> ; area <span style="color: #339933"> = myrec. <span style="color: #006633"> calculateArea <span style="color: #009900"> ( <span style="color: #009900"> ) <span style="color: #339933"> ; <span style="color: #003399"> System. <span style="color: #006633"> out. <span style="color: #006633"> println <span style="color: #009900"> ( <span style="color: #0000ff"> "The area of Rectangle2: " <span style="color: #339933"> +area <span style="color: #009900"> ) <span style="color: #339933"> ; <span style="color: #009900"> }
<span style="color: #009900"> } </span style="color: #009900"> </span style="color: #009900"> </span style="color: #339933"> </span style="color: #009900"> </span style="color: #339933"> </span style="color: #0000ff"> </span style="color: #009900"> </span style="color: #006633"> </span style="color: #006633"> </span style="color: #003399"> </span style="color: #339933"> </span style="color: #009900"> </span style="color: #009900"> </span style="color: #006633"> </span style="color: #339933"> </span style="color: #339933"> </span style="color: #009900"> </span style="color: #cc66cc"> </span style="color: #cc66cc"> </span style="color: #009900"> </span style="color: #003399"> </span style="color: #000000;font-weight: bold"> </span style="color: #339933"> </span style="color: #003399"> </span style="color: #339933"> </span style="color: #009900"> </span style="color: #339933"> </span style="color: #0000ff"> </span style="color: #009900"> </span style="color: #006633"> </span style="color: #006633"> </span style="color: #003399"> </span style="color: #339933"> </span style="color: #009900"> </span style="color: #009900"> </span style="color: #006633"> </span style="color: #339933"> </span style="color: #339933"> </span style="color: #009900"> </span style="color: #009900"> </span style="color: #003399"> </span style="color: #000000;font-weight: bold"> </span style="color: #339933"> </span style="color: #003399"> </span style="color: #339933"> </span style="color: #000066;font-weight: bold"> </span style="color: #009900"> </span style="color: #009900"> </span style="color: #009900"> </span style="color: #009900"> </span style="color: #003399"> </span style="color: #009900"> </span style="color: #000066;font-weight: bold"> </span style="color: #000000;font-weight: bold"> </span style="color: #000000;font-weight: bold"> </span style="color: #009900"> </span style="color: #000000;font-weight: bold"> </span style="color: #009900"> </span style="color: #009900"> </span style="color: #339933"> </span style="color: #339933"> </span style="color: #000000;font-weight: bold"> </span style="color: #009900"> </span style="color: #009900"> </span style="color: #009900"> </span style="color: #000066;font-weight: bold"> </span style="color: #009900"> </span style="color: #339933"> </span style="color: #339933"> </span style="color: #339933"> </span style="color: #339933"> </span style="color: #009900"> </span style="color: #009900"> </span style="color: #000066;font-weight: bold"> </span style="color: #000066;font-weight: bold"> </span style="color: #009900"> </span style="color: #003399"> </span style="color: #009900"> </span style="color: #339933"> </span style="color: #cc66cc"> </span style="color: #339933"> </span style="color: #339933"> </span style="color: #cc66cc"> </span style="color: #339933"> </span style="color: #009900"> </span style="color: #009900"> </span style="color: #009900"> </span style="color: #003399"> </span style="color: #339933"> </span style="color: #000066;font-weight: bold"> </span style="color: #339933"> </span style="color: #000066;font-weight: bold"> </span style="color: #009900"> </span style="color: #003399"> </span style="color: #000000;font-weight: bold"> </pre class="java" style="font-family:monospace">
Explanation:
Here, method play() of superclass waka_waka_song is overridden by subclasses Spotify and Amazon Music.
Output:
Conclusion
Hope the above article helped you build a concrete understanding of polymorphism in Java. For such educational content, stay tuned to Shiksha Online. And if you have any queries feel free to reach us at the link below.
FAQs
Why use Mathutil in the Java?
In the example code I provided, MathUtils is just the name of a simple Java class that I created to demonstrate the concept of method overloading. In real-world scenarios, classes like MathUtils are often used to group related utility methods together for easy access and code organization. For example, the Math class in Java's standard library provides a collection of mathematical utility methods, such as Math.max(), Math.min(), and Math.sqrt(), that can be used by Java programs to perform common mathematical operations. By organizing related methods into a utility class like MathUtils, developers can more easily find and use these methods in their code.
Can we rename Mathutil to anything or is it predefined like a keyword?
You can name your Java classes almost anything you want, as long as the name meets certain rules and conventions. For example, class names should start with an uppercase letter and use camel case, where the first letter of each word (except the first word) is capitalized. In the case of the MathUtils class I created in the example code, I chose that name to indicate that it contains utility methods for performing mathematical operations. However, you could certainly choose a different name for your own utility class based on its purpose or functionality. Just be sure to choose a name that accurately reflects the purpose of the class, and follows the standard naming conventions for Java classes.
What is Super Class in Java?
In Java, a superclass is like a parent class that can have many child classes. The parent class holds common properties and methods that can be shared by its child classes. Think of it like a family, where the parent is the superclass and the children are the child classes. The children can inherit traits from their parent, just like child classes can inherit properties and methods from their superclass.

Experienced AI and Machine Learning content creator with a passion for using data to solve real-world challenges. I specialize in Python, SQL, NLP, and Data Visualization. My goal is to make data science engaging an... Read Full Bio